IntroductionSimcenter Amesim can model a wide variety of multi-physics systems. In some cases, our system may include a number of repetitive components or subsystems in which the typical copy/paste capability is not sufficient to speed up the sketching and parameterization. A simple example can be a series of thermal capacitance, with different parameters for each; we can copy-paste the component in the sketch, but all the connections and different parameters must be set manually.
For that reason (and many others) Simcenter Amesim provides a complete set of API functions to write short programs in higher abstraction level languages, like Python, C, and Visual Basic for Applications, that automate interaction with models.
These functions are particularly designed for automating tasks, such as setting or getting parameter values, running temporal simulations, and post-processing results variables. These tasks may be combined to build small or larger applications that, for example:
- create models from scratch
- add, move, rotate, and connect components on the sketch
- assign submodels to components
- compile a model
- set run parameters
...
Application cases
The following models are cases in which this method can be used.
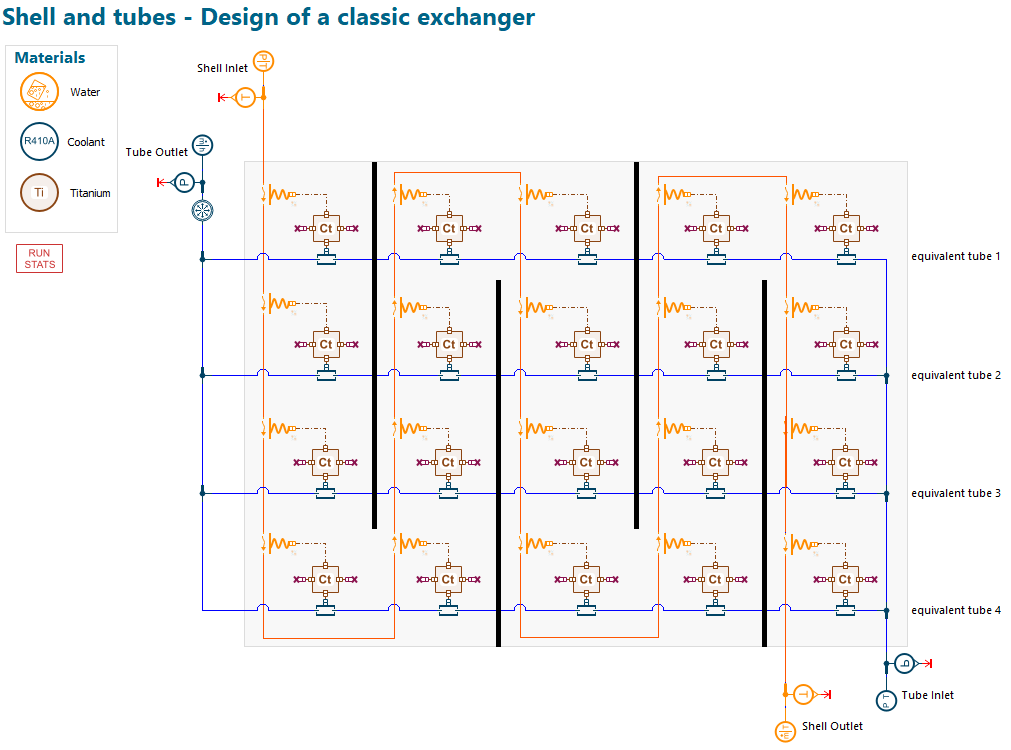

Exhaust Line with counter pressure and thermal exchanges
Battery pack air cooling with temperature distribution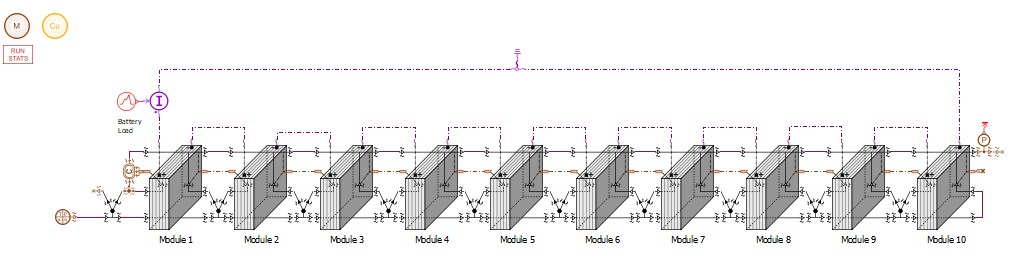
As seen, all of them consist of component sets repeated throughout the sketch. If each set shares the same parameters there is no problem with simply copying them. But, suppose that each one differs in one or more parameters or submodel types; or suppose that each set must be repeated tens or hundreds of times, even in the case that they all share the same parameters, the connections must be done manually, and would take hours to complete.
Example: Battery Pack for Air/Gas Cooling
To illustrate the basic principle of the algorithm used here, a battery pack model will be created using a typical distributive approach, this means, modeling each cell with a set of thermal capacitances and equivalent cell circuits (simple, advanced, electrochemical, or ultracapacitor); connected by thermal conductances and pneumatic flow volumes for the gas cooling, as shown below

Our main goal is to allow the user to specify the number of cells in X and Y directions, set the parameters for entire or partial rows/columns, and then automatically create the battery pack, as shown below (12x16 pack including case).
Find the python file in the attachments at the top. Its main procedure is explained and listed below
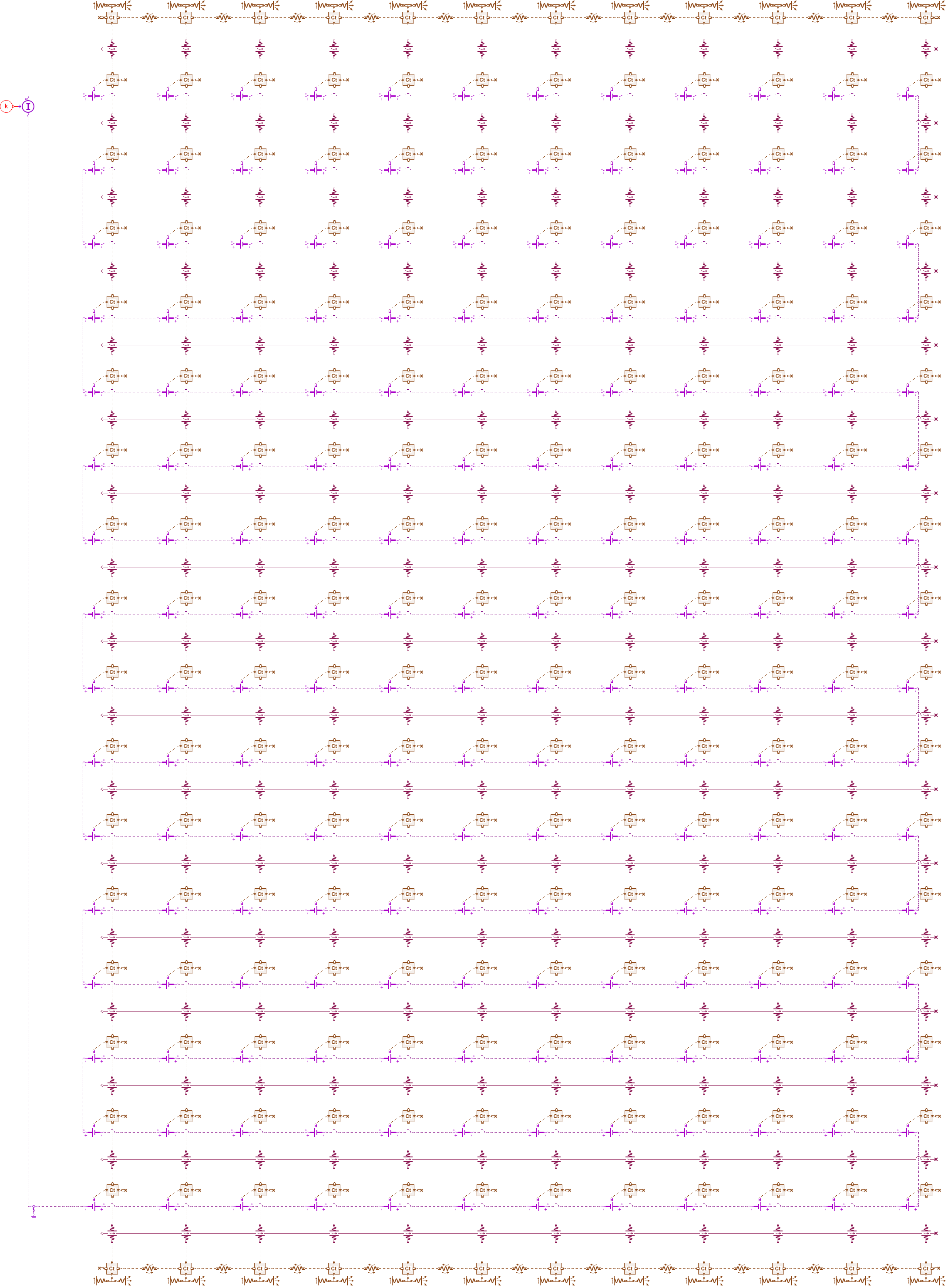
1. Import libraries
As with every python code, we start by importing the modules needed
_________________________________________________________________________________________________________________
import os, amesim, subprocess, sys
if 'ame_apy' not in sys.modules:
try:
from ame_apy import *
except ImportError:
print('Unable to import Simcenter Amesim API module.\nCheck the AME environment variable.')_________________________________________________________________________________________________________________
os: provides a portable way of using operating system dependent functionality
amesim: set of API functions
subprocess: allows to spawn new processes and obtain their return codes.
sys: provides functions and variables used to manipulate different parts of the Python runtime environment
2. Set up the Amesim environment and create the systemThe
AMEInitAPI() function must be called before doing anything else. It takes a Simcenter Amesim license token until
AMECloseAPI has been called.
_________________________________________________________________________________________________________________
# Set up the API.
AMEInitAPI()
# Change the working directory
os.chdir('C:/AMETest')
# Create new "BatteryPack" system
AMECreateCircuit('BatteryPack')_________________________________________________________________________________________________________________ 3. Add componentsAs mentioned, the main objective is to create the battery pack, based on the number of cells in the X and Y directions specified by the user.
Each Amesim API sketching function is pointed to the component alias, which must be unique for each function. We can take advantage of this rule and use it as our main algorithm, applying the Python string
replace() method inside a for loop definition, as follows.
_________________________________________________________________________________________________________________
# Specify the number of cells in x and y directions
X = 2
Y = 4
# Alias for TH capacitances
thc_alias = "cell_x_y"
for x in range(X):
for y in range(Y):
# Add TH capacitances
AMEAddComponent('th_c', thc_alias.replace('x',str(x)).replace('y',str(y)), (300+170*x, 300+170*y))
AMEChangeSubmodel(thc_alias.replace('x',str(x)).replace('y',str(y)), 'THC000', r'$AME\libth\submodels')_________________________________________________________________________________________________________________ A 2 by 4 matrix of thermal capacitances is created in the specified top-left pixel coordinates

We repeat the same algorithm for the rest of the components.
4. Cells concatenation for connection in seriesWe want to model a battery pack in series, therefore, we need to flip the battery cell equivalent circuits in each even column after placing them on the sketch, and their connections will depend on the specified columns to close the circuit. We specify said conditions with simple if statements.
_________________________________________________________________________________________________________________
# Alias for battery cell equivalent circuits
bat_alias = "bat_x_y"
for x in range(X):
for y in range(Y):
# Add battery cell equivalent circuits
AMEAddComponent('BatCellGene', bat_alias.replace('x',str(x)).replace('y',str(y)) , (250+170*x, 335+170*y))
AMERotateComponent(bat_alias.replace('x',str(x)).replace('y',str(y)))
AMEChangeSubmodel(bat_alias.replace('x',str(x)).replace('y',str(y)), 'ESSBATCSQS01', r'$AME\libess\submodels')
# Battery cells concatenation for connection in series
if y % 2 == 0:
AMEFlipComponent(bat_alias.replace('x',str(x)).replace('y',str(y))) _________________________________________________________________________________________________________________The cells are flipped for every even column
5. ConnectionsConnections follow the same logic, except for the electric ones, which, as mentioned, depend on the number of columns specified by the user.
_________________________________________________________________________________________________________________# Connect Battery Equivalent Circuits
for y in range(Y):
if y % 2 == 0:
for x in range(X-1):
AMEConnectTwoPortsWithLine(bat_alias.replace('x',str(x)).replace('y',str(y)), 0, bat_alias.replace('x',str(x+1)).replace('y',str(y)), 1, batline_alias.replace('x',str(x)).replace('y',str(y)), ())
elif y % 2 == 1:
for x in range(X-1):
AMEConnectTwoPortsWithLine(bat_alias.replace('x',str(x)).replace('y',str(y)), 1, bat_alias.replace('x',str(x+1)).replace('y',str(y)), 0, batline_alias.replace('x',str(x)).replace('y',str(y)), ())
for y in range(Y-1):
if y % 2 == 0:
AMEConnectTwoPortsWithLine(bat_alias.replace('x',str(X-1)).replace('y',str(y)), 0, bat_alias.replace('x',str(X-1)).replace('y',str(y+1)), 1, batcloselineR_alias.replace('x',str(x)).replace('y',str(y)), ())
elif y % 2 == 1:
AMEConnectTwoPortsWithLine(bat_alias.replace('x','0').replace('y',str(y)), 0, bat_alias.replace('x','0').replace('y',str(y+1)), 1, batcloselineL_alias.replace('x','0').replace('y',str(y)), ())_________________________________________________________________________________________________________________6. ParameterizationSimilarly, we use the "
replace()" function for parameterization. Modifying the for loop range we can set one or more parameters for entire rows/columns, or individual components. A couple of examples are included in the script
_________________________________________________________________________________________________________________# Set Cell parameters
for x in range(X):
AMESetParameterValue('geometry@'+thc_alias.replace('x',str(x)).replace('y','0'), '2')
AMESetParameterValue('volume@'+thc_alias.replace('x',str(x)).replace('y','0'), '2')
# Set Air convections parameters
for y in range(Y+1):
AMESetParameterValue('hconvType2@'+air_alias.replace('x','0').replace('y',str(y)), '1')
AMESetParameterValue('hconvImposed2@'+air_alias.replace('x','0').replace('y',str(y)), '80')
AMESetParameterValue('hconvType2@'+air_alias.replace('x',str(X-1)).replace('y',str(y)), '1')
AMESetParameterValue('hconvImposed2@'+air_alias.replace('x',str(X-1)).replace('y',str(y)), '80')_________________________________________________________________________________________________________________7. Save and close the systemAdding (True) to the
AMECloseCircuit line enables the circuit to be saved before it is closed. Insert
AMESaveCircuit before
AMECloseCircuit to achieve the same result
_________________________________________________________________________________________________________________AMECloseCircuit(True)
AMECloseAPI()_________________________________________________________________________________________________________________Run the codeWe can start the file from a command line prompt, by typing
AMEPython [Filename].py:
If you start the file from within Python Command Interpreter, type exec(open("[Filename].py").read())