Simcenter Amesim provides a complete set of scripts to write short programs in higher abstraction level languages like Python, MATLAB, or Visual Basic Application that automate interaction with models. This guide gives an application example to be able to link our global parameters with an excel file in both directions: from Excel to Simcenter Amesim and from Simcenter Amesim to Excel. The basic principle is converting a list of dictionaries, which is the format that Simcenter Amesim reads a global parameters configuration, to rows and columns of a table, and vice-versa
Python Shell ScriptStart the Python shell script with one of these methods:
- Call the Python command from a command-line interface of your Operating System.
- In Simcenter Amesim, select Tools > Python command interpreter.
The following welcome message is displayed:
Figure 1: Python Command Interpreter displayed
Installing external modules to the Python Command Interpreter
Usually, when we want to export a large number of global parameters to excel we want to manipulate them using all the excel functions. Therefore, the best way to export them is to a .xls extension file. However, in this case, we need a python module that is not installed by default in the Amesim command interpreter. The easiest way to install it is by typing
_________________________________________________________________________________________
pip install xlwt
͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞
Normally,
pip is automatically installed if you are working in a virtual environment, using Python downloaded from python.org, or using Python that has not been modified by a redistributor to remove
ensurepip. If your Python environment does not have
pip installed, there are 2 mechanisms to install pip supported directly by pip’s maintainers:
_________________________________________________________________________________________
ensurepip
get-pip.py
͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞
If that didn't work, we can manually download the necessary files and place them into the corresponding Simcenter Amesim folder. Close the command interpreter and get the files from
https://pypi.org/project/xlwt/ in the section ’
Download Files’.
Select the
.tar.gz extension files.
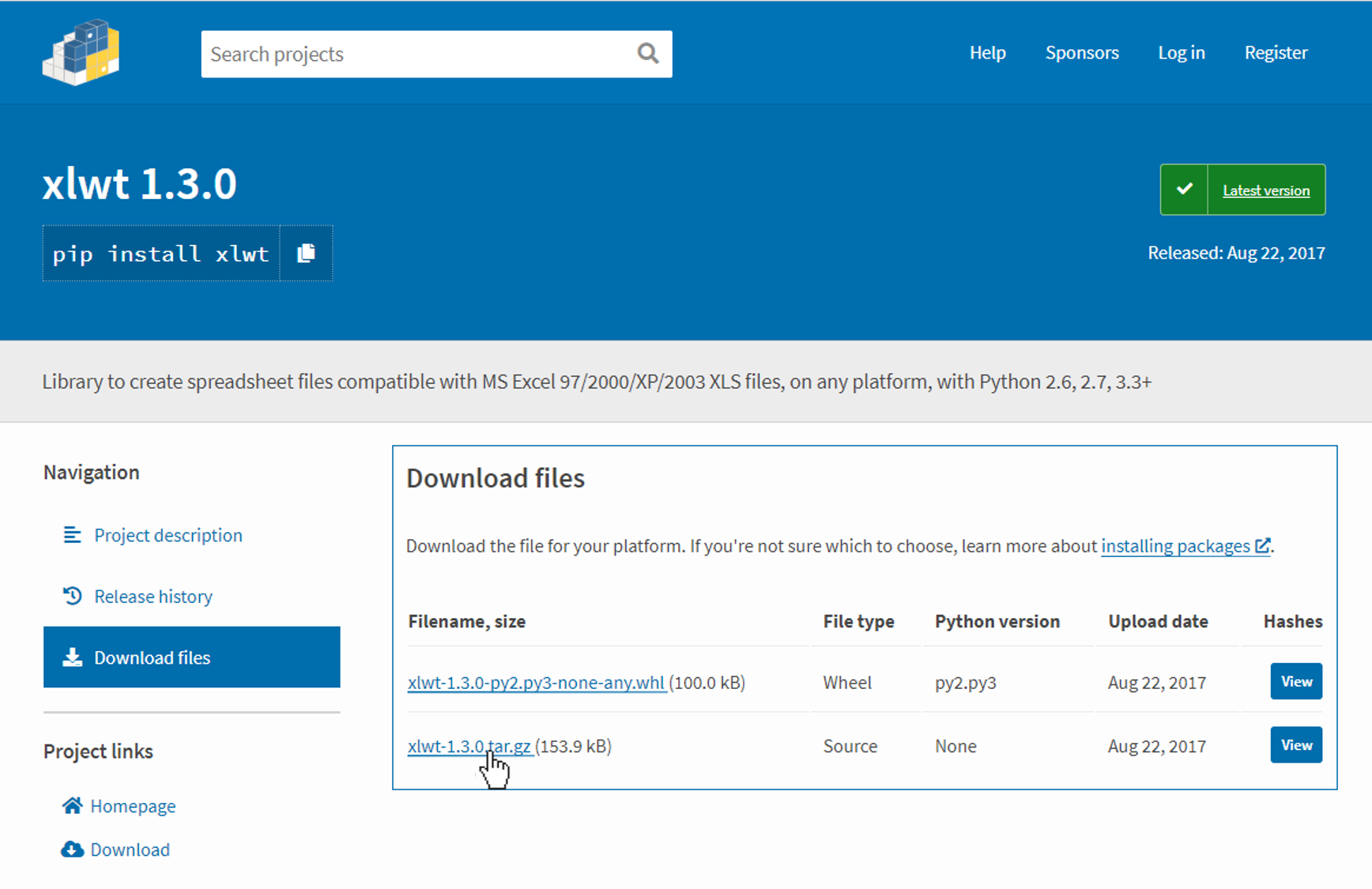
Figure 2.
xlwt module files, download.
Extract the downloaded file in an arbitrary path and copy the folder ’xlwt-1.3.0’, which has the main files (Figure 3). Is recommended to have a compression and management utility like WinRAR or WinZip
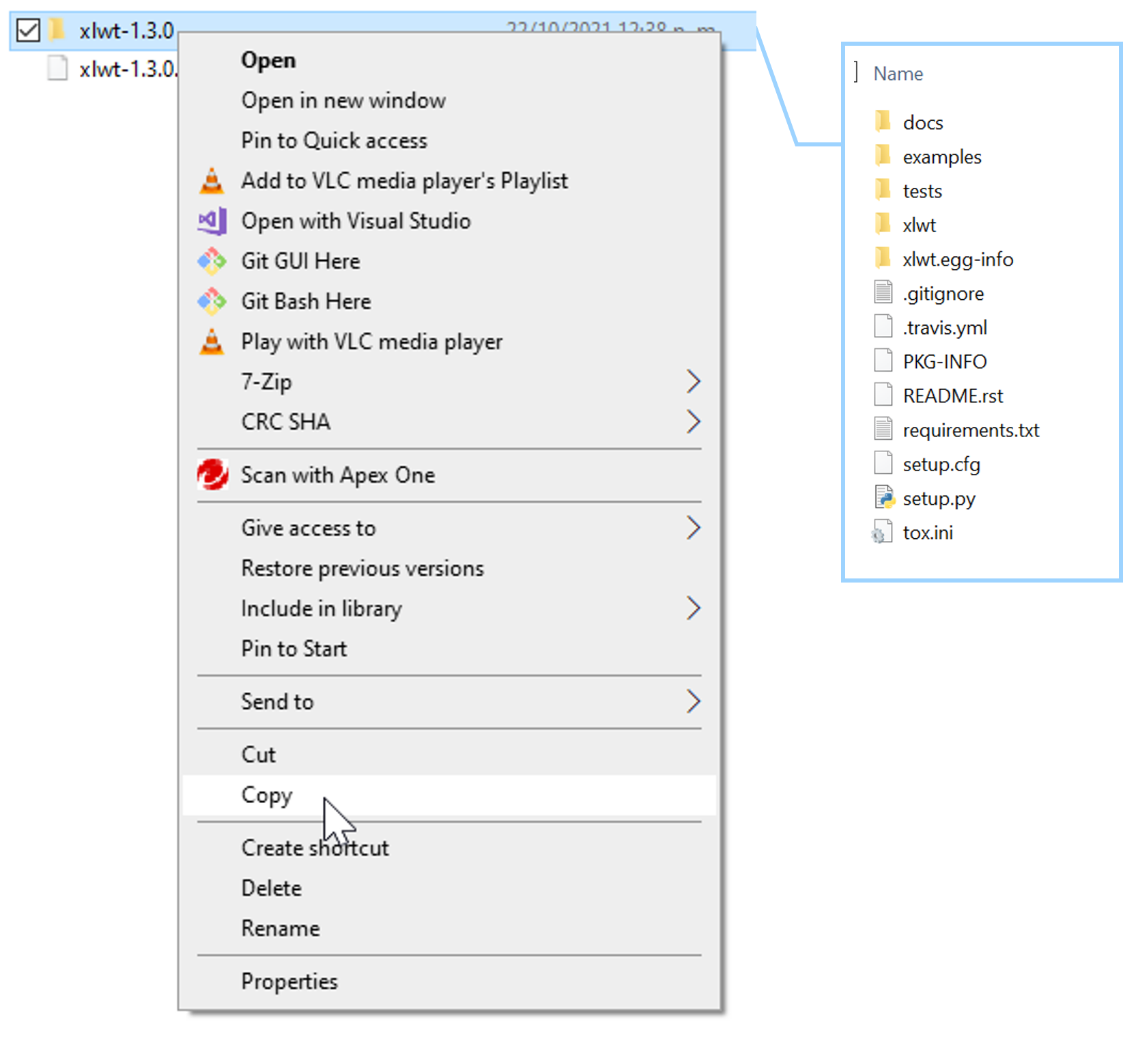
Figure 3.
xlwt module files, copy the main folder.
Paste it into the python site-packages folder of Simcenter Amesim, usually found in the path:
C:\Program Files\Simcenter\2021.1\Amesim\sys\python\win64\Lib\site-packages
Figure 4. xlwt module files, paste the main folder.
Note: Alternatively, you can get the folder in the attached zip file of this knowledge article.
For version 2021.2 we also need to paste the folders 'xlwt' and 'xlwt-1.3.0.dist-info' also provided in the attached file, since they were removed
Exporting Global Parameters to Excel File
For this example, we are going to use the demo system ’FlatTwin’. Get the system from the demos
1. Select Help > Get demo. The Choose Demo dialog box opens.
2. Open the Tutorials folder and select FlatTwin.ame.
3. Click on Copy and open. The system below is shown.
Figure 5. Flat Twin demo system
4. Go to Parameter mode and look at the six global parameters.
Figure 6. System Global Parameters
5. Start the Python shell script with one of the previously described methods and look at the current directory, this is the path where the Excel file is going to be created
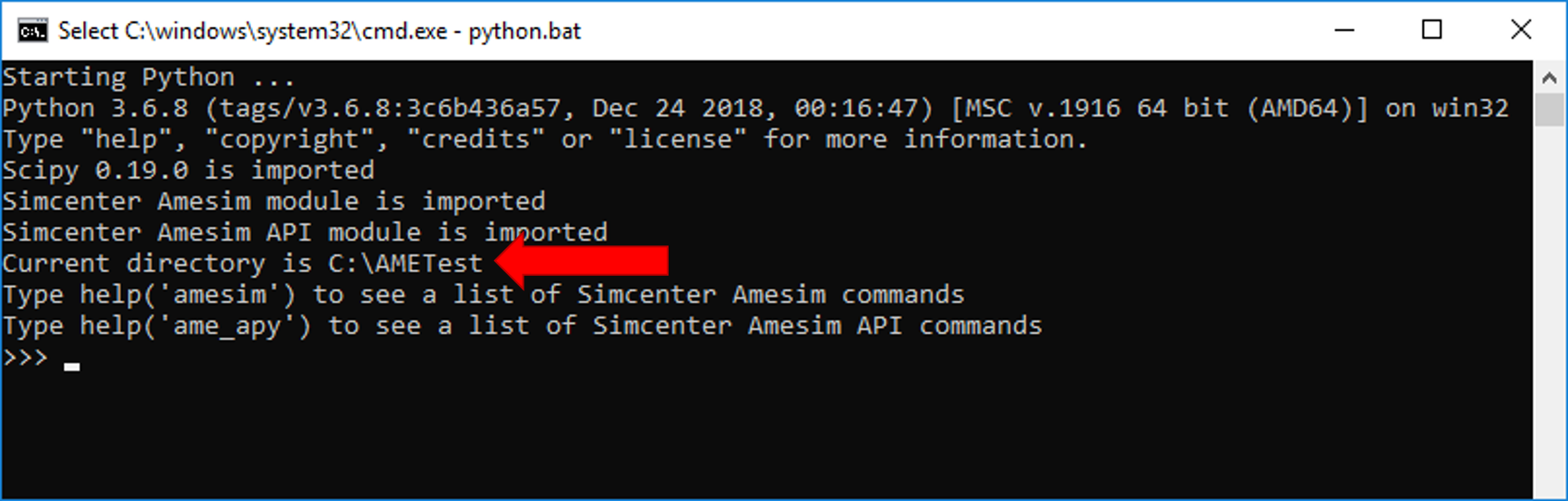
Figure 7. Python command interpreter
6. Type or copy/paste the following code and run it. Verify that the specified name
('FlatTwin') matches your opened model. That's the only line that we need to edit to run the code for other models. Also the generated file name
('data.xls')if desired.
_________________________________________________________________________________________
import xlwt
w = xlwt.Workbook()
ws = w.add_sheet('sheet1')
gp_list,ret_stat=amereadgp('FlatTwin')
columns = list(gp_list[0].keys())
for i, row in enumerate(gp_list):
for j, col in enumerate(columns):
ws.write(i, j, row[col])
w.save('data.xls')
͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞
7. A new .xls file has been created into the current directory, in this case C:\AMETest. Open the file, and verify if all parameters have been exported as desired. We can specify the type of value for each column in the first row based on the Global Parameter Setup of our Amesim model.
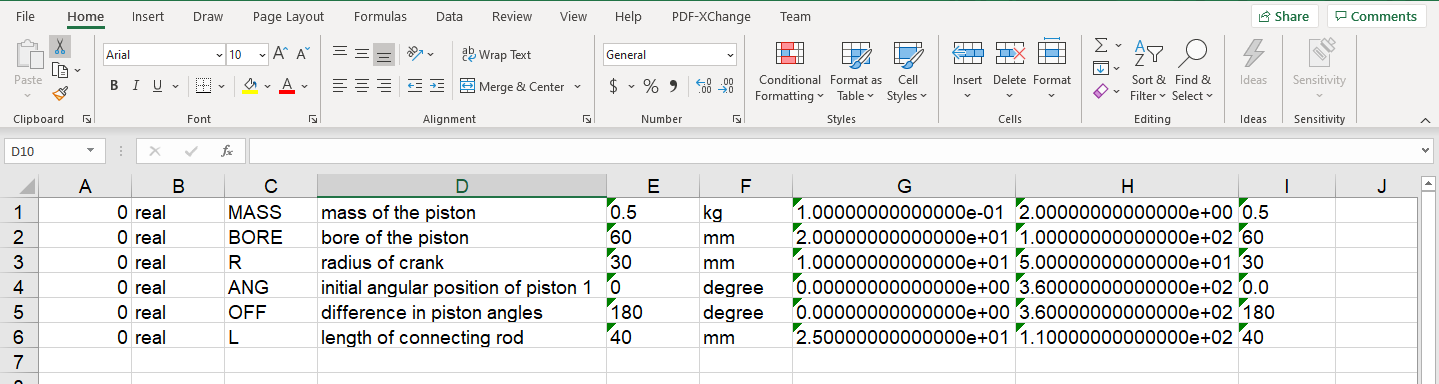
Figure 8. Excel data created
Importing Global Parameters from Excel FileThere are several ways to import parameters from excel. As we mentioned, the basic principle is converting the rows and columns of a table to a list of dictionaries, which is the format that Simcenter Amesim reads a .amegp file. To do this in a simple way we can convert the desired file to a .csv extension and use the
csv module, which is one of the default python modules. In this way, we avoid installing another one, like
xlrd,
openpyxl, or
pandas.
We can proceed as follows:
1. Open the desired file, and specify the key-value for each column, as seen below
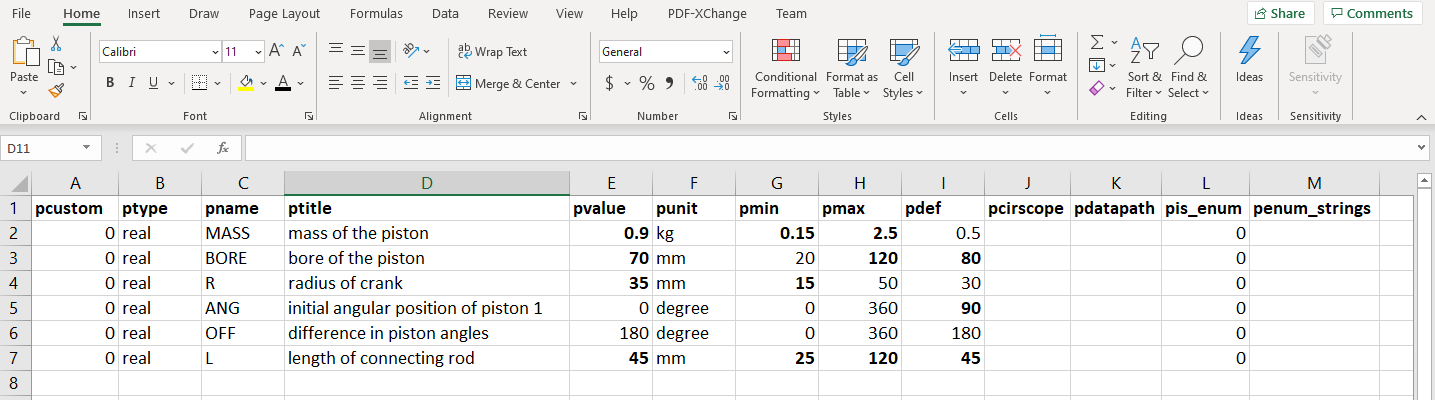
Figure 9. Data to export with key-value in the first row
We have changed some of the values (displayed in bold font) to notice the change after we import them.
Note: Each element of a global parameter list is described by a dictionary having the following key-value pairs:
- pcustom: '1' for parameters of customized objects; '0' otherwise
- ptype: 'integer', 'real' or 'text'
- pname: name of parameter
- ptitle: full title of parameter
- pvalue: value (as a string)
- pmin: min value (as a string, empty for 'text' type)
- pmax: max value (as a string, empty for 'text' type)
- pdef: default value (as a string)
- punit: unit for 'real' type, an empty string otherwise
- pcirscope: global parameter circuit scope id, empty for non custom parameters
- pdatapath: global parameter data path, empty for non custom parameters
Hence, this step is crucial since we are specifying the type of data that Amesim is going to insert into the Global
Parameter Setup.
2. Save the file as .csv
3. Start the Python shell script
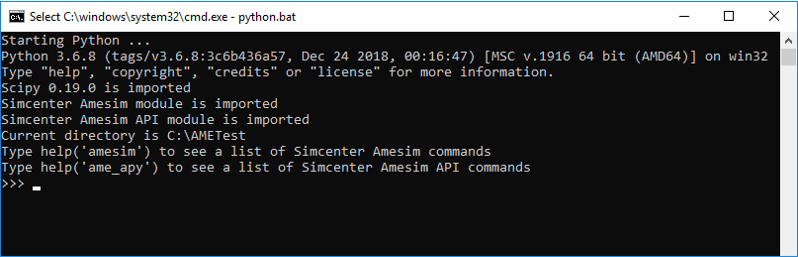
Figure 10. Python Command Interpreter
4. Type or copy/paste the following code and run it
_________________________________________________________________________________________
import csv
gp_list = []
filename ="data.csv"
with open(filename, 'r') as data:
for line in csv.DictReader(data):
gp_list.append(line)
amewritegp('FlatTwin', gp_list)
͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞ ͞
A new .amegp extension file has been created into the current directory. Open the Global Parameter Setup, delete the current parameters and load the new file.
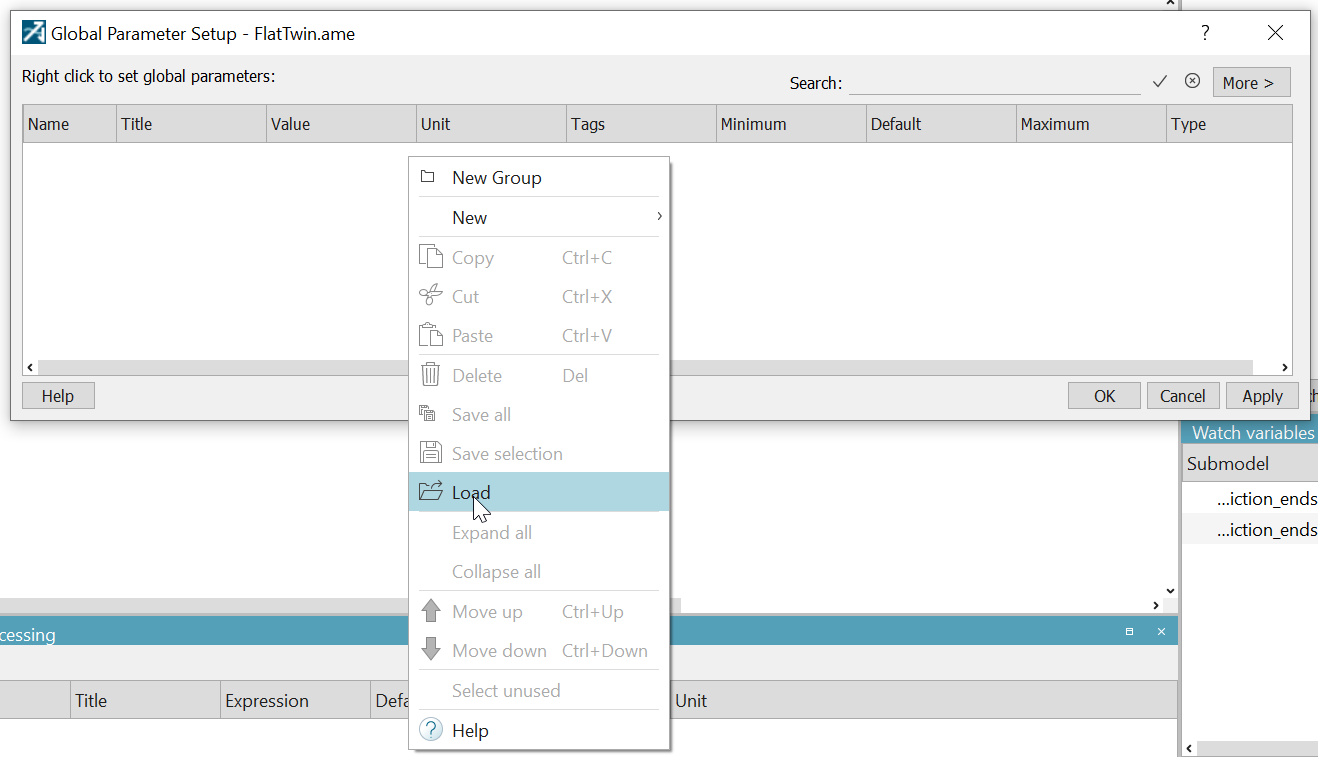

Figure 11. Load new Global Parameters file
The new Global Parameter Setup has been created